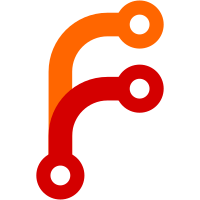
Commit 577fe9b
removed the debconf template for the option but the
maintainer scripts were still referencing it. This prevented the package
configuration from working.
145 lines
4.4 KiB
Bash
145 lines
4.4 KiB
Bash
#!/bin/bash
|
|
# postinst script for dump1090
|
|
#
|
|
# see: dh_installdeb(1)
|
|
|
|
set -e
|
|
|
|
# summary of how this script can be called:
|
|
# * <postinst> `configure' <most-recently-configured-version>
|
|
# * <old-postinst> `abort-upgrade' <new version>
|
|
# * <conflictor's-postinst> `abort-remove' `in-favour' <package>
|
|
# <new-version>
|
|
# * <postinst> `abort-remove'
|
|
# * <deconfigured's-postinst> `abort-deconfigure' `in-favour'
|
|
# <failed-install-package> <version> `removing'
|
|
# <conflicting-package> <version>
|
|
# for details, see http://www.debian.org/doc/debian-policy/ or
|
|
# the debian-policy package
|
|
|
|
NAME=dump1090-mutability
|
|
CONFIGFILE=/etc/default/$NAME
|
|
SRCCONFIGFILE=$CONFIGFILE
|
|
TEMPLATECONFIG=/usr/share/$NAME/config-template
|
|
CRONFILE=/etc/cron.d/$NAME
|
|
TEMPLATECRON=/usr/share/$NAME/cron-template
|
|
SEDSCRIPT=$CONFIGFILE.sed.tmp
|
|
|
|
subvar_raw() {
|
|
# $1 = config value
|
|
# $2 = config var name
|
|
|
|
if ! grep -Eq "^ *$2=" $SRCCONFIGFILE; then
|
|
# if not present in the config file, add it at the end
|
|
echo "\$a $2=$1" >> $SEDSCRIPT
|
|
else
|
|
# otherwise, replace the current value
|
|
echo "s@^ *$2=.*@$2=\"$1\"@" >>$SEDSCRIPT
|
|
fi
|
|
}
|
|
|
|
subvar() {
|
|
# $1 = db var name
|
|
# $2 = config var name
|
|
db_get $NAME/$1
|
|
subvar_raw "$RET" "$2"
|
|
}
|
|
|
|
subvar_yn() {
|
|
# $1 = db var name
|
|
# $2 = config var name
|
|
db_get $NAME/$1
|
|
if [ "$RET" = "true" ]; then subvar_raw "yes" "$2"; else subvar_raw "no" "$2"; fi
|
|
}
|
|
|
|
case "$1" in
|
|
configure)
|
|
. /usr/share/debconf/confmodule
|
|
|
|
# If we have no config file, start from the template.
|
|
# Avoid copying the template to the config file
|
|
# before substitution; this leaves an all-blank config
|
|
# in place if something fails, which causes problems
|
|
# on subsequent reconfiguration.
|
|
SKIPLINES=0
|
|
if [ ! -e $SRCCONFIGFILE ]; then
|
|
SRCCONFIGFILE=$TEMPLATECONFIG
|
|
SKIPLINES=4
|
|
fi
|
|
|
|
rm -f $SEDSCRIPT
|
|
|
|
subvar_yn auto-start START_DUMP1090
|
|
subvar run-as-user DUMP1090_USER
|
|
subvar log-file LOGFILE
|
|
subvar rtlsdr-device DEVICE
|
|
subvar rtlsdr-gain GAIN
|
|
subvar rtlsdr-ppm PPM
|
|
subvar_yn rtlsdr-oversample OVERSAMPLE
|
|
subvar_yn decode-fixcrc FIX_CRC
|
|
subvar_yn decode-phase-enhance PHASE_ENHANCE
|
|
subvar decode-lat LAT
|
|
subvar decode-lon LON
|
|
subvar decode-max-range MAX_RANGE
|
|
subvar net-http-port HTTP_PORT
|
|
subvar net-ri-port RAW_INPUT_PORT
|
|
subvar net-ro-port RAW_OUTPUT_PORT
|
|
subvar net-bi-port BEAST_INPUT_PORT
|
|
subvar net-bo-port BEAST_OUTPUT_PORT
|
|
subvar net-sbs-port SBS_OUTPUT_PORT
|
|
subvar net-heartbeat NET_HEARTBEAT
|
|
subvar net-out-size NET_OUTPUT_SIZE
|
|
subvar net-out-interval NET_OUTPUT_INTERVAL
|
|
subvar net-buffer NET_BUFFER
|
|
subvar net-bind-address NET_BIND_ADDRESS
|
|
subvar stats-interval STATS_INTERVAL
|
|
subvar json-dir JSON_DIR
|
|
subvar json-interval JSON_INTERVAL
|
|
subvar json-location-accuracy JSON_LOCATION_ACCURACY
|
|
subvar_yn log-decoded-messages LOG_DECODED_MESSAGES
|
|
subvar extra-args EXTRA_ARGS
|
|
|
|
tail -n +$SKIPLINES < $SRCCONFIGFILE | sed -f $SEDSCRIPT > $CONFIGFILE.tmp
|
|
if [ -e $CONFIGFILE ]; then
|
|
chown --reference=$CONFIGFILE $CONFIGFILE.tmp
|
|
chmod --reference=$CONFIGFILE $CONFIGFILE.tmp
|
|
fi
|
|
mv -f $CONFIGFILE.tmp $CONFIGFILE
|
|
rm $SEDSCRIPT
|
|
|
|
db_get $NAME/run-as-user
|
|
RUNAS="$RET"
|
|
if ! getent passwd "$RUNAS" >/dev/null
|
|
then
|
|
adduser --system --home /usr/share/$NAME --no-create-home --quiet "$RUNAS"
|
|
fi
|
|
|
|
# create log if missing; change ownership if needed so the cronjob works
|
|
db_get $NAME/log-file
|
|
touch $RET
|
|
chown $RUNAS $RET
|
|
|
|
# this config file has changed a few times, restart lighttpd to make sure we
|
|
# have the latest version
|
|
if [ -e /etc/lighttpd/conf-enabled/89-dump1090.conf ]; then
|
|
echo "Restarting lighttpd.." >&2
|
|
invoke-rc.d lighttpd restart || echo "Warning: lighttpd failed to restart." >&2
|
|
fi
|
|
;;
|
|
|
|
abort-upgrade|abort-remove|abort-deconfigure)
|
|
;;
|
|
|
|
*)
|
|
echo "postinst called with unknown argument \`$1'" >&2
|
|
exit 1
|
|
;;
|
|
esac
|
|
|
|
# dh_installdeb will replace this with shell code automatically
|
|
# generated by other debhelper scripts.
|
|
|
|
#DEBHELPER#
|
|
|
|
if [ "$1" = "configure" ]; then db_stop; fi
|
|
exit 0
|